Python has had awesome string formatters for many years but the documentation on them is far too theoretic and technical. With this site we try to show you the most common use-cases covered by the old and new style string formatting API with practical examples.
All examples on this page work out of the box with with Python 2.7, 3.2, 3.3, 3.4, and 3.5 without requiring any additional libraries.
Further details about these two formatting methods can be found in the official Python documentation:
Introduction to Python 3 Cheat Sheet. Python is a high-level programming language that was designed by Guido Van Rossum and developed by the Python software foundation in 1990. It has a paradigm of Object-oriented, imperative and procedural reflective. The parent company of python is a Python. Python Cheat Sheet Christina Costello February 20, 2020 09:24. Formatting string date field to a date data type where the field has NULLS and blanks in it. This cheat sheet explores what Python is used for and how it compares to other programming languages, and provides resources for learning the language. Other format works as intuitively with pandas. Reshaping Data –Change the layout of a data set M. A F M. A pd.melt(df) Gather columns into rows. Df.pivot(columns='var', values='val') Spread rows into columns. Pd.concat(df1,df2) Append rows of DataFrames pd.concat(df1,df2, axis=1) Append columns of DataFrames df.sortvalues('mpg').
If you want to contribute more examples, feel free to create a pull-request on Github!
Table of Contents:
Basic formatting
Simple positional formatting is probably the most common use-case. Use itif the order of your arguments is not likely to change and you only havevery few elements you want to concatenate.
Since the elements are not represented by something as descriptive as aname this simple style should only be used to format a relatively smallnumber of elements.
Old
New
Output
Old
New
Output
With new style formatting it is possible (and in Python 2.6 even mandatory)to give placeholders an explicit positional index.
This allows for re-arranging the order of display without changing thearguments.
This operation is not available with old-style formatting.
New
Output
Value conversion
The new-style simple formatter calls by default the __format__()
method of an object for its representation. If you just want to render theoutput of str(...)
or repr(...)
you can use the !s
or !r
conversionflags.
In %-style you usually use %s
for the string representation but there is%r
for a repr(...)
conversion.
Setup
Old
New
Output
In Python 3 there exists an additional conversion flag that uses the outputof repr(...)
but uses ascii(...)
instead.
Setup
Old
New
Output
Padding and aligning strings
By default values are formatted to take up only as many characters asneeded to represent the content. It is however also possible to define thata value should be padded to a specific length.
Unfortunately the default alignment differs between old and new styleformatting. The old style defaults to right aligned while for new styleit's left.
Align right:
Old
New
Output
Old
New
Output
Again, new style formatting surpasses the old variant by providing morecontrol over how values are padded and aligned.
You are able to choose the padding character:
This operation is not available with old-style formatting.
New
Output
This operation is not available with old-style formatting.
New
Output
When using center alignment where the length of the string leads to an uneven split of the padding characters the extra character will be placed on the right side:
This operation is not available with old-style formatting.
New
Output
Truncating long strings
Inverse to padding it is also possible to truncate overly long valuesto a specific number of characters.
The number behind a .
in the format specifies the precision of theoutput. For strings that means that the output is truncated to thespecified length. In our example this would be 5 characters.
Old
New
Output
Combining truncating and padding
It is also possible to combine truncating and padding:
Old
New
Output
Numbers
Of course it is also possible to format numbers.
Integers:
Old
New
Output
Old
New
Output
Padding numbers
Similar to strings numbers can also be constrained to a specific width.
Old
New
Output
Again similar to truncating strings the precision for floating pointnumbers limits the number of positions after the decimal point.
For floating points the padding value represents the length of the completeoutput. In the example below we want our output to have at least 6characters with 2 after the decimal point.
Old
New
Output
For integer values providing a precision doesn't make much sense and isactually forbidden in the new style (it will result in a ValueError).
Old
New
Output
Signed numbers
By default only negative numbers are prefixed with a sign. This can bechanged of course.
Old
New
Output
Use a space character to indicate that negative numbers should be prefixedwith a minus symbol and a leading space should be used for positive ones.
Old
New
Output
Old
New
Output
New style formatting is also able to control the position of the signsymbol relative to the padding.
This operation is not available with old-style formatting.
New
Output
New
Output
Named placeholders
Both formatting styles support named placeholders.
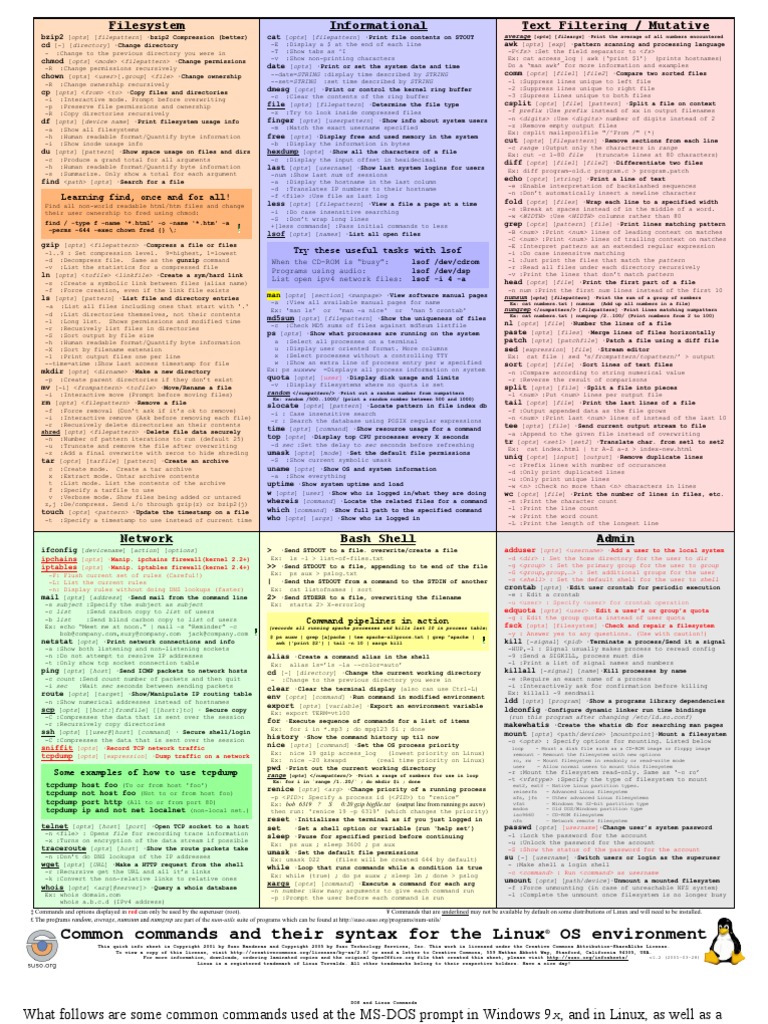
Setup
Old
New
Output
This operation is not available with old-style formatting.
New
Output
Getitem and Getattr
New style formatting allows even greater flexibility in accessing nesteddata structures.
It supports accessing containers that support __getitem__
like forexample dictionaries and lists:
This operation is not available with old-style formatting.
Setup
New
Output
Setup
New
Output
As well as accessing attributes on objects via getattr()
:
This operation is not available with old-style formatting.
Setup
New
Output
Both type of access can be freely mixed and arbitrarily nested:
This operation is not available with old-style formatting.
Setup
New
Output
Datetime
New style formatting also allows objects to control their ownrendering. This for example allows datetime objects to be formatted inline:
This operation is not available with old-style formatting.
Setup
New
Output
Parametrized formats
Additionally, new style formatting allows all of the components ofthe format to be specified dynamically using parametrization. Parametrizedformats are nested expressions in braces that can appear anywhere in theparent format after the colon.
Old style formatting also supports some parametrization but is much morelimited. Namely it only allows parametrization of the width and precisionof the output.
Parametrized alignment and width:
This operation is not available with old-style formatting.
New
Output
Old
New
Output
Old
New
Output
The nested format can be used to replace any part of the formatspec, so the precision example above could be rewritten as:
This operation is not available with old-style formatting.
New
Output
The components of a date-time can be set separately:
This operation is not available with old-style formatting.
Setup
New
Output
The nested formats can be positional arguments. Position dependson the order of the opening curly braces:
Python Command Cheat Sheet
This operation is not available with old-style formatting.
New
Output
And of course keyword arguments can be added to the mix as before:
This operation is not available with old-style formatting.
New
Output
Custom objects
The datetime example works through the use of the __format__()
magicmethod. You can define custom format handling in your own objects byoverriding this method. This gives you complete control over the formatsyntax used.
This operation is not available with old-style formatting.
Setup
New
Output
Do you want to learn Python but you’re overwhelmed and you don’t know where to start? Learn with Python cheat sheets! They compress the most important information in an easy-to-digest 1-page format.
Here’s the new Python cheat sheet I just created—my goal was to make it the world’s most concise Python cheat sheet!
While working as a researcher in distributed systems, Dr. Christian Mayer found his love for teaching computer science students.
To help students reach higher levels of Python success, he founded the programming education website Finxter.com. He’s author of the popular programming book Python One-Liners (NoStarch 2020), coauthor of the Coffee Break Python series of self-published books, computer science enthusiast, freelancer, and owner of one of the top 10 largest Python blogs worldwide.
His passions are writing, reading, and coding. But his greatest passion is to serve aspiring coders through Finxter and help them to boost their skills. You can join his free email academy here.
Python Format Cheat Sheet
Related Posts
